I have a feature class with parsed address fields and I need a single concatenated field. Some of the addresses have directionals like “N” or “S”, some have units, and some don’t. A simple expression will litter the field with extra spaces.
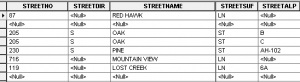
Here is a short Python snippet that uses string formatting & the Update Cursor to populate a field with only the relevant data for each record.
# concatenate parsed address fields without extra spaces | |
import arcpy | |
featureClass = "c:\\myfakepath\\data.gdb\\fc" | |
# create an expression to find records where the STREETNO field is not blank | |
# this indicates an address to be concatenated | |
expression = "STREETNO IS NOT NULL" | |
# create a list of the fields to be concatenated | |
# (the last field in the list is the field to be concatenated in to) | |
fields = ["STREETNO","STREETDIR","STREETNAME","STREETSUF","STREETUNIT","STREETADDR"] | |
# create an update cursor limited to the fields in the fields list, and by the expression | |
try: | |
with arcpy.da.UpdateCursor(featureClass,fields,expression) as cursor: | |
for row in cursor: | |
if not row[1]: ## if streetdir is null | |
exp = "{0} {1}".format(str(row[0]),row[2]) ## streetno + streetname | |
else: ## streetdir is not null | |
exp = "{0} {1} {2}".format(row[0],row[1],row[2])## streetno + streetdir + streetname | |
if row[3]: ## if streetsuf is not null | |
exp += " {0}".format(row[3]) ## add streetsuf to exp | |
if row[4]: ## if streetunit is not null | |
exp += " {0}".format(row[4]) ## add streetunit to exp | |
row[5] = exp ## set streetaddr field = exp | |
cursor.updateRow(row) | |
except Exception as e: | |
error = "Failed: concat fields\n {0} {1}".format(arcpy.GetMessages(), str(e)) | |
print(error) |
Fields returned by the cursor are accessed by index in the order they are listed in the list, so row[0] refers to the value in the STREETNO field, row[1] is the STREETDIR field, and so on.
A series of conditionals tests whether the “extra” fields have content, and successively builds the final concatenation expression using string formatting. Here I am using numbers for placeholders in the string formatting substitution, but it is not strictly necessary.
Line 25 setsĀ the appropriateĀ field (which has an index of 5) to this expression, and then line 26 calls “updateRow” to make the change.
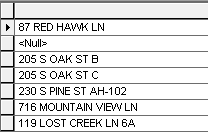
ArcGIS Desktop documentation: